reCAPTCHA: Balancing User Experience with Security
Exploring available reCAPTCHA methods for protection against spam
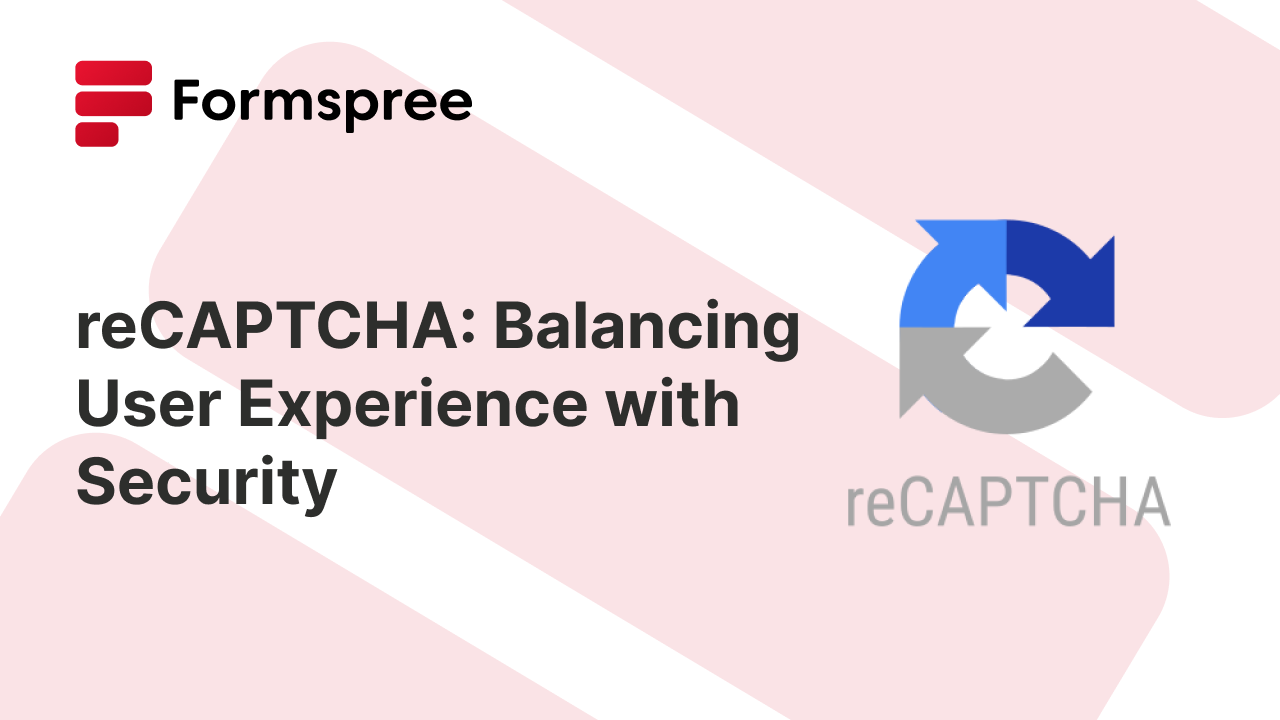
reCAPTCHA is a free service from Google that helps websites identify real users from bots. It presents users with challenges that are easy for humans but difficult for automated programs to solve, preventing spam activity, which helps to keep your website secure and functioning smoothly. However, there are a number of reCAPTCHA methods you can use to protect your web forms against spam.
This guide will list some of the different reCAPTCHA methods by which you can implement reCAPTCHA in your web apps, particularly when building forms using Formspree.
reCAPTCHA Methods
For Formspree users, there are four main types of reCAPTCHA methods, each offering a different balance between security and user experience.
We will explore these options in depth to help you choose the right one for your application.
reCAPTCHA v2 (Interstitial)
This is one of the easiest ways to set up Google reCAPTCHA in your Formspree forms. Instead of integrating a reCAPTCHA script in your webpage, you only need to enable the reCAPTCHA checkbox in the Settings tab for your form on the Formspree dashboard:
Upon submitting the form, the user will be redirected to an interstitial page that contains only the reCAPTCHA checkbox. Clicking the checkbox verifies that the user is a human. It may also include an optional image selection task where users identify specific objects in a grid of images.
This is how the page will look like:
When enabling reCAPTCHA in the Formspree dashboard, make sure to keep the Custom reCAPTCHA Key field empty if you want to use the interstitial reCAPTCHA page.
As you’ve seen, this method is super simple to set up. However, the interstitial page is branded with Formspree’s branding. This method is great when you are looking to get done with the reCAPTCHA setup quickly, but adds an additional step in the form submission process, which might be seen as a clunky UX. Additionally, this method involves a redirect to the interstitial page and should only be used with HTML forms. This approach isn’t appropriate for JavaScript forms that use AJAX.
reCAPTCHA v2 (Checkbox)
This is the most recognizable version of reCAPTCHA, with a checkbox that users must tick to confirm they’re human. This is the same reCAPTCHA you saw above in the interstitial method, but in this method, you can integrate the reCAPTCHA checkbox on the same page as the form. As a result, this approach can be used with either HTML or JavaScript (AJAX) forms.
Implementing this is quite simple.
You need to head over to the Google reCAPTCHA admin console to generate a set of reCAPTCHA v2 (checkbox) keys and then plug it into your app as shown below:
<html>
<head>
<!-- Add the following script -->
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
</head>
<body>
<form method="POST" action="https://formspree.io/f/{form_id}">
<input type="email" name="email" placeholder="Your email">
<textarea name="message" placeholder="Test Message"></textarea>
<!-- Add the following div to render the reCAPTCHA checkbox -->
<div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div>
<button type="submit">Send Test</button>
</form>
</body>
<html>
Remember to replace "YOUR_SITE_KEY"
with your actual site key obtained from the Google reCAPTCHA Admin console. Also, you need to enable the reCAPTCHA checkbox in the Settings tab for your form on the Formspree dashboard and provide the secret key in the Custom reCAPTCHA key field.
This will render the reCAPTCHA v2 checkbox on the screen as soon as the page loads. If you’d prefer to load and invoke the challenge programmatically, you can take a look at Google’s documentation.
This method offers moderate security against bots as some advanced bots can still figure out the test. Also, it requires at least one click from the user to complete the challenge which might degrade the user experience of your forms.
reCAPTCHA v2 (Invisible)
Google also offers reCAPTCHA v2 as an invisible check on the user activity instead of having to ask the users to manually complete a challenge. This is similar to the checkbox version, however, instead of a click on a checkbox, Google looks for a click on a button to trigger the reCAPTCHA verification. So, it is very easy to implement in your forms with something as simple as adding a few properties to the submit button.
To implement this method, you need to head over to the Google reCAPTCHA admin console to generate a set of reCAPTCHA v2 (invisible) keys and then plug it into your app as shown below:
<html>
<head>
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
<script>
function onSubmit(token) {
document.getElementById("my-form").submit();
}
</script>
</head>
<body>
<form id="my-form" method="POST" action="https://formspree.io/f/{form_id}">
<input type="email" name="email" placeholder="Your email">
<textarea name="message" placeholder="Test Message"></textarea>
<button class="g-recaptcha" data-sitekey="YOUR_SITE_KEY" data-callback="onSubmit">Send Test</button>
</form>
</body>
</html>
Remember to replace “YOUR_SITE_KEY” with your actual site key obtained from the Google reCAPTCHA Admin console. You will also need to enable the reCAPTCHA checkbox in the Settings tab for your form on the Formspree dashboard and provide the secret key in the Custom reCAPTCHA key field.
This will bind the reCAPTCHA v2 invisible challenge to the submit button conveniently. If you’d prefer to load and invoke the challenge programmatically, you can take a look at Google’s documentation.
This method also offers moderate security as it relies on analyzing user behavior in the background to identify bots. However, there are no additional clicks that the user needs to do, so the user experience is unaffected by the reCAPTCHA challenge.
reCAPTCHA v3
Finally, Google offers (and recommends) reCAPTCHA v3 as a seamless CAPTCHA challenge for users. reCAPTCHA v3 analyzes various user interactions on your website, from mouse movements and clicks to form-filling behavior. Based on this analysis, it assigns a score between 0 (very likely bot) and 1 (very likely human).
This score reflects the risk of a user being a bot. v3 helps build a great user experience by not interrupting users with challenges. It works as a risk assessment tool in the background that allows you to take appropriate action based on the calculated score. This balance between a smooth user flow and security makes v3 a popular choice for many websites.
V3 provides the most robust bot protection and also supports Google’s reCAPTCHA Enterprise, providing a more customizable model for large businesses.
Implementing reCAPTCHA v3 is somewhat simple compared to the other options you’ve seen so far. First of all, you need to head over to the Google reCAPTCHA admin console to generate a set of reCAPTCHA v3 keys and then plug it into your app as shown below:
<html>
<head>
<script src="https://www.google.com/recaptcha/api.js"></script>
<script>
function onSubmit() {
document.getElementById("myForm").submit()
}
</script>
</head>
<body>
<form id="myForm" action="https://formspree.io/f/{form_id}" method="POST">
<input type="email" name="email" placeholder="Your email">
<textarea name="message" placeholder="Test Message"></textarea>
<button class="g-recaptcha" data-sitekey="YOUR_SITE_KEY" data-callback='onSubmit'
data-action='submit'>Submit</button>
</form>
</body>
</html>
Remember to replace "YOUR_SITE_KEY"
with your actual site key obtained from the Google reCAPTCHA Admin console. Make sure to enable the reCAPTCHA checkbox in the Settings tab for your form on the Formspree dashboard and provide the secret key in the Custom reCAPTCHA key field.
This will bind the reCAPTCHA v3 challenge to the submit button. Alternatively, if you want more control over when reCAPTCHA runs, you can take a look at Google’s documentation.
This method offers the best bot detection out of all the methods discussed here. The score assessment happens in the background, so there are no added hiccups in the user experience.
One key point to consider is its reliance on extensive data collection. v3 analyzes various user interactions to generate the reCAPTCHA score, raising questions about compliance with regulations like GDPR that emphasize data privacy. This collection of user behavior data, without the ability to opt out, can be worrying for privacy-conscious users who don’t want their online activities tracked and analyzed.
Integration Considerations
Here’s a quick rundown of all the four methods against some key factors:
Setup Difficulty:
- Easiest: v2 Interstitial requires no code changes but relies on Formspree settings.
- Moderate: Other options require minimal code changes. They also need additional scripting for programmatic challenge invocation.
User Experience:
- Smoothest: v3 offers a seamless experience with no user interaction. v2 Invisible avoids disrupting user flow with an initial challenge.
- Disruptive: v2 Checkbox requires users to click a checkbox and might prompt additional challenges.
- Most Disruptive: v2 Interstitial presents a full-fledged, possibly branded challenge interrupting the user flow.
Security vs. Privacy:
- Moderate Security, Good Privacy: v2 Checkbox and Interstitial offer moderate security but require explicit user interaction, potentially disrupting user experience.
- Low Security, Good Privacy: v2 Invisible analyzes user behavior for bot detection, offering a better UX but potentially less secure than the other options.
- High Security, Low Privacy: v3 provides the highest potential security through risk scoring, but relies heavily on user data collection which can raise privacy concerns.
Consider your priorities. If user experience is the most important factor for you, v3 or v2 Invisible are good options. If privacy is a bigger concern and user experience is less critical, v2 Checkbox or Interstitial might be better. Finally, v3 offers the best balance of security and user experience but comes with potential privacy drawbacks.
Conclusion
Choosing the ideal reCAPTCHA method boils down to your specific needs. If a smooth user flow with the highest security is crucial, reCAPTCHA v3 offers a seamless solution with risk-based security. However, it comes at the cost of user data collection, a potential privacy concern. For a similar experience that collects less data, v2 Invisible provides a good compromise, analyzing user behavior subtly. v2 checkbox offers the highest security without collecting as much data as the other options but comes at the cost of user experience. Finally, Formspree’s interstitial CAPTCHA provides a super simple integration within Formspree forms with high security.
While the future of reCAPTCHA is uncertain, the current efforts on leveraging AI for risk analysis with minimal disruption is a promising path. As bots become more sophisticated, reCAPTCHA’s ability to adapt through AI advancements will be crucial. This also highlights the importance of using reCAPTCHA v3, which offers the latest improvements in this ongoing battle. By staying updated with the latest version, you’ll be better equipped to handle the ever-evolving challenges of automated threats.