Top Methods for Server-Side Validation with Examples
Learn the role of server-side validation in web development and understand its importance in ensuring security, data integrity, and user experience.
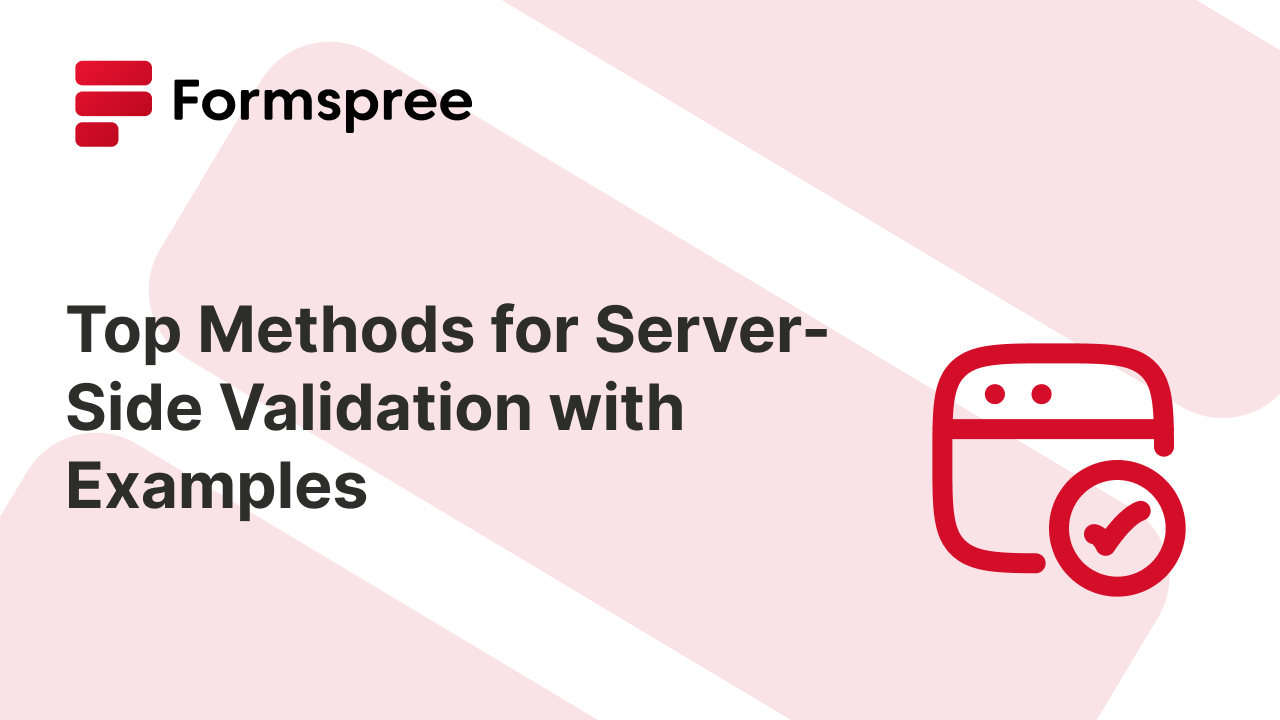
Form validation is an important aspect of any web form, ensuring that the data users submit through it meets the required standards before it reaches your server. While client side validation provides a quick way to catch errors, it’s not foolproof and can be bypassed. This is where server-side validation comes into play, acting as the final checkpoint to enforce data integrity and security.
In this article, you’ll learn about the key methods for implementing server-side validation, complete with practical examples. Whether you’re using popular backend frameworks, creating custom validation functions, or using middleware, this guide will equip you with the tools to create robust and secure validation processes in your applications.
Why Do You Need Server-side Validation?
Server side validation helps to maintain the security and integrity of your web applications. Unlike client side validation which can be easily bypassed, server side validation ensures that all data submitted to your server is properly checked and conforms to the required standards. This is important for avoiding incomplete or “garbage” input, and for preventing common security vulnerabilities such as SQL injection and cross-site scripting (XSS), which can compromise your application if unchecked.
Additionally, server side validation provides consistency across different clients and browsers. It ensures that no matter where the data originates from, it is uniformly processed and validated according to your application’s rules. This consistency is especially important in applications dealing with complex data validation or sensitive information, as it guarantees that the data meets your requirements before any further processing occurs.
Top Methods for Implementing Server-Side Validation
Now that you understand why server side validation is important, it’s time to explore the top methods for implementing it effectively. In this section, we’ll explore various approaches, ranging from utilizing popular backend frameworks to creating custom validation functions, and even leveraging middleware and schema-based validation techniques
Validation Using Popular Backend Frameworks
Using popular backend frameworks for server-side validation is one of the easiest ways. Most “full stack” web frameworks support tools and libraries that simplify the process of validating user input, allowing you to enforce data constraints, prevent malicious data, and handle errors effectively.
For example, in Node.js with Express, you can use the validator
library to implement validation directly within your route handlers. This library provides a wide range of functions to validate and sanitize inputs, ensuring that user-submitted data meets your application’s requirements.
Here’s a basic example of how to use validator
in an Express route:
const express = require('express');
const validator = require('validator');
const app = express();
app.use(express.json());
app.post('/register', (req, res) => {
const { displayName, firstName, lastName, company, email } = req.body;
let errors = [];
// Validate display name (minimum length 5 and alphanumeric only)
if (!validator.isLength(displayName, { min: 5 })) {
errors.push('Chosen display name must be at least 5 characters long');
}
if (!validator.isAlphanumeric(displayName)) {
errors.push('Display name can consist of alphabets and numbers only');
}
// Validate email
if (!validator.isEmail(email)) {
errors.push('Please enter a valid email address');
}
// Validate names
if (validator.isEmpty(firstName)) {
errors.push('Please enter a first name');
}
if (validator.isEmpty(lastName)) {
errors.push('Please enter a last name');
}
// Validate company
if (validator.isEmpty(company)) {
errors.push('Please enter your company\'s name');
}
// If there are validation errors, return them to the user
if (errors.length > 0) {
return res.status(400).json({ errors });
}
// If validation passed, continue with registration process
res.send('Registration successful!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Here’s what would happen if you choose a display name that does not pass the validation requirements (and enter an invalid email as well):
Similarly, in Django, Python’s popular web framework, you can leverage Django’s built-in form and field validation features. These tools let you define validation rules within your models or forms, ensuring that all data stored in your database meets your application’s requirements.
Ruby on Rails also offers robust validation options through Active Record, its Object-Relational Mapping (ORM) system. With Active Record, you can easily define validation rules for your database models, ensuring that only valid data is persisted. These frameworks not only simplify the validation process but also integrate conveniently with other aspects of your application, making it easier to maintain and scale your validation logic as your project grows.
Schema-Based Validation
Schema-based validation is a powerful and flexible method for enforcing data integrity in server-side applications. By defining a schema—a blueprint that specifies the structure, types, and constraints of your data—you can automate the validation process, ensuring that all incoming data conforms to the expected format. This approach is particularly useful in applications where data validation rules are complex or need to be consistently applied across multiple endpoints.
In the Node.js ecosystem, libraries like Joi provide robust schema-based validation capabilities. With Joi, you can define schemas for your data, specifying the types, required fields, minimum and maximum lengths, patterns, and more. These schemas can then be used to validate incoming data, automatically checking for compliance and generating informative error messages if the data doesn’t match the schema.
Here’s a basic example using Joi in an Express application:
const express = require('express');
const Joi = require('joi');
const app = express();
app.use(express.json());
const registrationSchema = Joi.object({
firstName: Joi.string().required(),
lastName: Joi.string().required(),
displayName: Joi.string().min(5).alphanum().required(),
email: Joi.string().email().required(),
company: Joi.string().required(),
});
app.post('/register', (req, res) => {
const { error } = registrationSchema.validate(req.body);
if (error) {
return res.status(400).json({ errors: error.details.map(detail => detail.message) });
}
res.send('Registration successful!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Here’s how the validation result looks:
In this example, the schema defines the validation rules for the firstName
, lastName
, displayName
, email
, and company
fields. If the data does not meet these requirements, Joi automatically generates detailed error messages, allowing you to handle validation errors efficiently. Schema-based validation with tools like Joi not only simplifies the validation process but also makes your code more maintainable and scalable.
Custom Validation Functions
Custom validation functions offer a customized approach to server side validation, allowing you to implement specific rules that built-in validation methods in frameworks or libraries may not cover. This method is particularly useful when you need to implement unique business logic or complex data structures that require specialized validation beyond the scope of standard validators.
In a Node.js/Express application, you can create custom validation functions within your route handlers or as separate modules, depending on the complexity and reusability of the logic. These functions give you full control over the validation process and enable you to enforce precise rules and handle edge cases effectively.
Here’s an example of how to implement a custom validation function in an Express route:
const express = require('express');
const app = express();
app.use(express.json());
function validateDisplayName(displayName) {
if (displayName.length < 5) {
return 'The display name must be at least 5 characters long';
}
if (/[^A-Za-z0-9]+/.test(displayName)) {
return 'The display name must only contain alphabets and numbers';
}
return null;
}
app.post('/register', (req, res) => {
const { displayName, firstName, lastName, company, email } = req.body;
let errors = [];
// Custom displayName validation
const displayNameError = validateDisplayName(displayName);
if (displayNameError) {
errors.push(displayNameError);
}
// Other validations...
if (errors.length > 0) {
return res.status(400).json({ errors });
}
res.send('Registration successful!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Here’s how a custom validation function for the display name field works:
In this example, the validateDisplayName
function checks if the display name meets specific criteria, such as minimum length and containing only alphanumeric characters. If the display name fails any of these checks, an appropriate error message is returned. By setting up custom validation functions like this, you can enforce specific business rules and ensure that all data meets your application’s unique requirements. This approach enhances the flexibility and precision of your validation logic, making your application more robust and secure.
Using Middlewares
Using middleware for server-side validation is an efficient way to centralize and manage your validation logic in web applications. Middleware functions in frameworks like Express allow you to intercept and process requests before they reach your route handlers, making them ideal for implementing validation checks.
With middleware, you can create reusable validation logic that can be applied across multiple API routes, ensuring consistency and reducing code duplication. This approach is especially useful in larger applications where the same validation rules need to be enforced in multiple parts of the application.
Here’s an example of how to use middleware for validation in an Express application:
const express = require('express');
const validator = require('validator');
const app = express();
app.use(express.json());
const validateRegistration = (req, res, next) => {
const { displayName, firstName, lastName, company, email } = req.body;
let errors = [];
// Validate username (minimum length 5)
if (!validator.isLength(displayName, { min: 5 })) {
errors.push('Chosen display name must be at least 5 characters long');
}
if (!validator.isAlphanumeric(displayName)) {
errors.push('Display name can consist of alphabets and numbers only');
}
// Validate email
if (!validator.isEmail(email)) {
errors.push('Please enter a valid email address');
}
// Validate names
if (validator.isEmpty(firstName)) {
errors.push('Please enter a first name');
}
if (validator.isEmpty(lastName)) {
errors.push('Please enter a last name');
}
// Validate company
if (validator.isEmpty(company)) {
errors.push('Please enter your company\'s name');
}
// If there are validation errors, return them to the user
if (errors.length > 0) {
return res.status(400).json({ errors });
}
next(); // Proceed to the next middleware or route handler if validation passes
}
app.post('/register', validateRegistration, (req, res) => {
res.send('Registration successful!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Here’s how the validation works:
In this example, the validateRegistration
middleware function is applied to the /register
route. It checks the validity of the firstName
, lastName
, displayName
, email
, and company
fields before passing control to the route handler. If the validation fails, it returns a 400 Bad Request
response with error messages; otherwise, it proceeds to the next middleware or the final route handler. By using middleware for validation, you can create clean, maintainable code that ensures consistent data validation across your application.
Using Formspree
If you are looking to set up forms on your website and want to do away with all the hassle of setting up the backend logic for validating form data on the server side and then storing it, let alone triggering emails and other workflows after submission, you might be better off with Formspree.
Formspree is a form backend service that simplifies form handling by offering built-in server-side validation. This feature can help you streamline your form processing while ensuring that all submissions meet the required data integrity standards.
Setting up Formspree with server-side validation in an HTML app is straightforward. Once you’ve created a form in HTML, you can use AJAX to handle form submissions and let Formspree manage the validation and backend processing.
Here’s an example of how to set it up:
<form id="contact-form">
<input type="email" name="email" placeholder="Your email" required>
<input type="text" name="name" placeholder="Your name" required>
<textarea name="message" placeholder="Your message" required></textarea>
<button type="submit">Send</button>
</form>
<script>
document.getElementById('contact-form').addEventListener('submit', function(event) {
event.preventDefault();
const formData = new FormData(this);
fetch('https://formspree.io/f/your-form-id', {
method: 'POST',
body: formData,
headers: {
'Accept': 'application/json'
}
}).then(response => {
if (response.ok) {
alert('Form submitted successfully!');
} else {
response.json().then(data => {
if (data.errors) {
alert('Validation failed: ' + data.errors.map(error => error.message).join(', '));
}
});
}
});
});
</script>
In this example, the form data is sent to Formspree using AJAX. You can now go to your Formspree dashboard, create a new form using the + Add New button at the top left, and go to the Workflows tab:
Here, you can define custom validation rules for your form. Try clicking on the red + Add New link in the VALIDATION section and adding rules for name and message:
For each field, Formspree will ask you to first choose its type:
And then depending on the type, you can set up validation rules:
Once you have set up the rules, here’s what the workflow page will look like:
Now when you try submitting the form, the form will be processed successfully only if the input data satisfies all the validation requirements you have set up on Formspree. If any validation rules fail, Formspree returns detailed error messages, which you can display to the user. This setup ensures that your forms are securely processed with minimal effort, making Formspree a convenient solution for managing form submissions.
Key Considerations for Server Side Validation
When implementing server side validation, there are several key considerations to keep in mind to ensure your application is both secure and efficient. First and foremost, all user inputs must be validated rigorously. This means checking for proper data types, formats, and constraints to prevent malicious data from entering your system. Client side input validation is critical in protecting against vulnerabilities like SQL injection and cross-site scripting (XSS), which can be exploited if data is not properly sanitized.
In addition to security, consider the performance implications of server-side validation. While thorough validation is necessary, it is also important to optimize these checks to avoid unnecessary overhead. This might involve prioritizing the most critical validations or simplifying complex validation logic to improve performance without sacrificing security.
Another important aspect is to handle invalid data gracefully. When a user submits incorrect or malformed data, provide clear and informative error messages that help them correct their input. This not only improves the user experience but also reduces the likelihood of repeated errors.
Finally, consistency in validation rules across your application is crucial. Ensure that the same validation logic is applied uniformly, regardless of where the data is processed. This consistency helps maintain data integrity and makes your validation logic easier to maintain and scale as your application grows.
Final Thoughts
In this article, we’ve highlighted the importance of server side validation in ensuring the security, integrity, and consistency of web applications. We explored various methods to implement server-side validation, including using backend frameworks, custom functions, middleware, and schema-based validation, each providing practical ways to safeguard your application.
As you integrate these validation techniques into your projects, remember that server-side validation is very important for building secure and reliable applications.
Ready to enhance your forms with built-in server-side validation? Try out Formspree today and streamline your validation process effortlessly.