Optimize Form Handling with jQuery Submit Form
Learn how to optimize form handling in your web apps using jQuery’s submit() method for seamless submissions and improved user experience
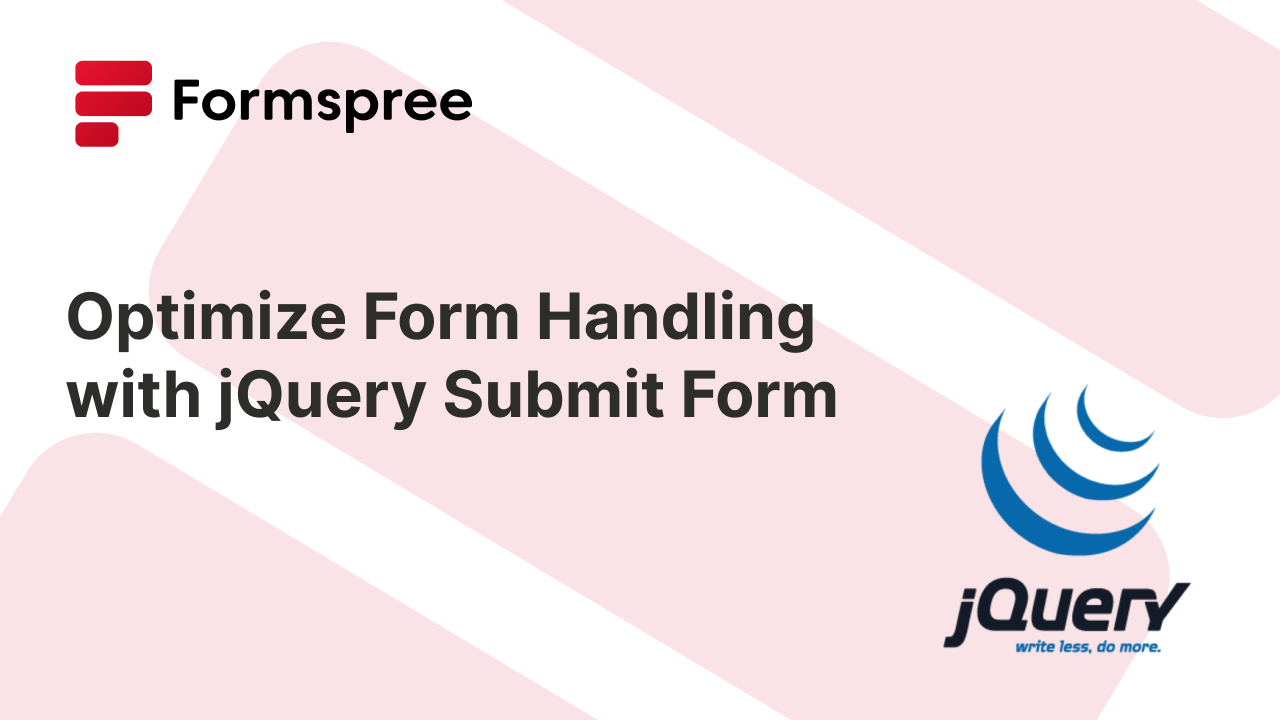
Handling form submissions is a very important aspect of web development, and understanding how jQuery’s submit()
method works can help you customize your HTML forms’s submission if you are using jQuery.
The now-deprecated submit()
method in jQuery allowed developers to handle the submit event programmatically, offering more flexibility than relying on a form’s default action. Whether you’re customizing the behavior of an HTML form with an input type="submit"
button or trying to catch a submit event triggered by an enter key, understanding jQuery’s submit method and the submit event can help you do form submissions with confidence.
In this article, we’ll dive into how jQuery’s submit event works, when to use it, and how to use it.
How jQuery’s submit Event Works
When you submit an HTML form, either by clicking the submit button or through any other method, jQuery’s submit
event is triggered. jQuery allows developers to bind event handlers to this event to execute custom behavior when a form is submitted. For starters, by using event.preventDefault()
, you can intercept and cancel the default action of the HTML form to handle custom form submission logic. You can then line up any set of steps to follow before you submit the form contents by yourself.
Another upside of using jQuery submit
is its ability to handle both synchronous and asynchronous form submissions. Synchronous submission involves using the default action triggered by a button type="submit"
or the enter key. This triggers the submit
event and any submit event handlers are triggered.
Asynchronous submission, on the other hand, uses AJAX requests to send form data without refreshing the page. This approach offers a slightly better experience for the users, especially with complex forms or multi-step submission workflows. jQuery code helps to simplify this process through methods like serialize()
and ajax()
that help in quickly serializing form data and initiating AJAX requests to the server.
When to Use jQuery’s submit
jQuery’s submit
is particularly useful in scenarios where you need precise control over form submission. Unlike the default action of an HTML form, submit
allows you to intercept and customize the form’s behavior. One common use case of this can be to validate form data before submission. For example, you might want to check if the data in your input fields meet specific requirements before allowing the form to proceed. Using the submit
event, you can bind an event handler to perform these validations and display error messages if necessary. This can help users correct issues before submitting the form, improving the quality of submissions.
Another scenario is submitting forms asynchronously using AJAX, as mentioned before. By combining submit
with jQuery’s ajax()
method, you can send form data to the server without reloading the page. This approach is especially beneficial for dynamic forms, where content or form elements are added or modified based on user interaction. For instance, a multi-step form can use the submit button to trigger data validation and an AJAX request for each step.
Compared to other jQuery methods like click()
or change()
, submit
is better suited for capturing the overall form submission process, especially when managing multiple form elements or handling the enter key for submission.
How to use jQuery’s submit()
While jQuery’s submit()
method was once widely used for handling form submission, it has been deprecated in jQuery 3.3 in favor of more uniform methods like .on()
for binding submit event handlers and .trigger()
for programmatic submissions. A possible reason) behind this could be that browsers and HTML standards keep adding new events, and creating and maintaining dedicated functions for these isn’t quite sustainable from jQuery’s perspective. The .on()
and .trigger()
methods were already present in jQuery to handle event processing, and they can perfectly handle the submit
event as well.
Using Formspree with jQuery submit
Formspree simplifies form handling by providing a backend to manage form submissions without requiring server-side code. By combining jQuery’s ability to intercept the submit
event with an AJAX request, you can send form data directly to Formspree.
Here’s an example of how to intercept a form’s submit
event and send the data to Formspree using $.ajax()
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Validation with jQuery and Formspree</title>
<script src="<https://code.jquery.com/jquery-3.1.1.js>"></script>
<script src="<https://cdn.jsdelivr.net/npm/jquery-validation@1.19.5/dist/jquery.validate.js>"></script>
<link rel="stylesheet" href="./styles.css" />
</head>
<body>
<form id="myForm">
<div class="fieldset">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
</div>
<div class="fieldset">
<label for="email">Email:</label>
<input type="email" id="email" name="email">
</div>
<button type="submit">Submit</button>
</form>
<script>
$('#myForm').on('submit', function(event) {
event.preventDefault(); // Prevent the default action
const formData = $(this).serialize(); // Extract and serialize form data
$.ajax({
url: '<https://formspree.io/f/{form_id}>', // Provide the URL to the forms backend
type: 'POST',
data: formData,
dataType: 'json',
success: function(response) {
console.log('Form submitted successfully');
},
error: function() {
alert('Error submitting form');
}
});
});
</script>
</body>
</html>
In the code above, you intercept the default action of a submit button or the enter button, serialize the form data, and send it to the Formspree servers using an AJAX request.
Submitting the Form Programmatically
To trigger the submit event programmatically, you can use the .trigger()
method in the following way:
$('form').trigger('submit');
This is helpful when submitting a form dynamically, for example, when a specific condition is met in your application.
Error Handling and Debugging
When working with the jQuery submit
event, you should always check for issues like missing form elements or improperly configured AJAX requests in the error
handler or a try-catch statement. You can use console.log()
statements to debug event propagation and ensure you call event.preventDefault()
to stop the form’s default action before implementing your custom logic.
Additionally, you should use tools like browser developer consoles to inspect AJAX requests and responses. Handling errors gracefully, such as displaying user-friendly error messages can provide a seamless user experience and create a reliable form submission process.
Conclusion
Understanding jQuery’s submit
and related methods like .on()
and .trigger()
enables developers to create dynamic, efficient, and user-friendly forms. Event handling, AJAX integration, form data serialization, and error management allow you to customize form submissions to fit diverse application needs. Whether validating form elements, handling multi-step workflows, or improving the user experience with seamless server communication, these tools offer flexibility and control.