A Mini Guide to HTML Form Action
Learn how to use the HTML form action attribute to control form submission and enhance user interactions on your website.
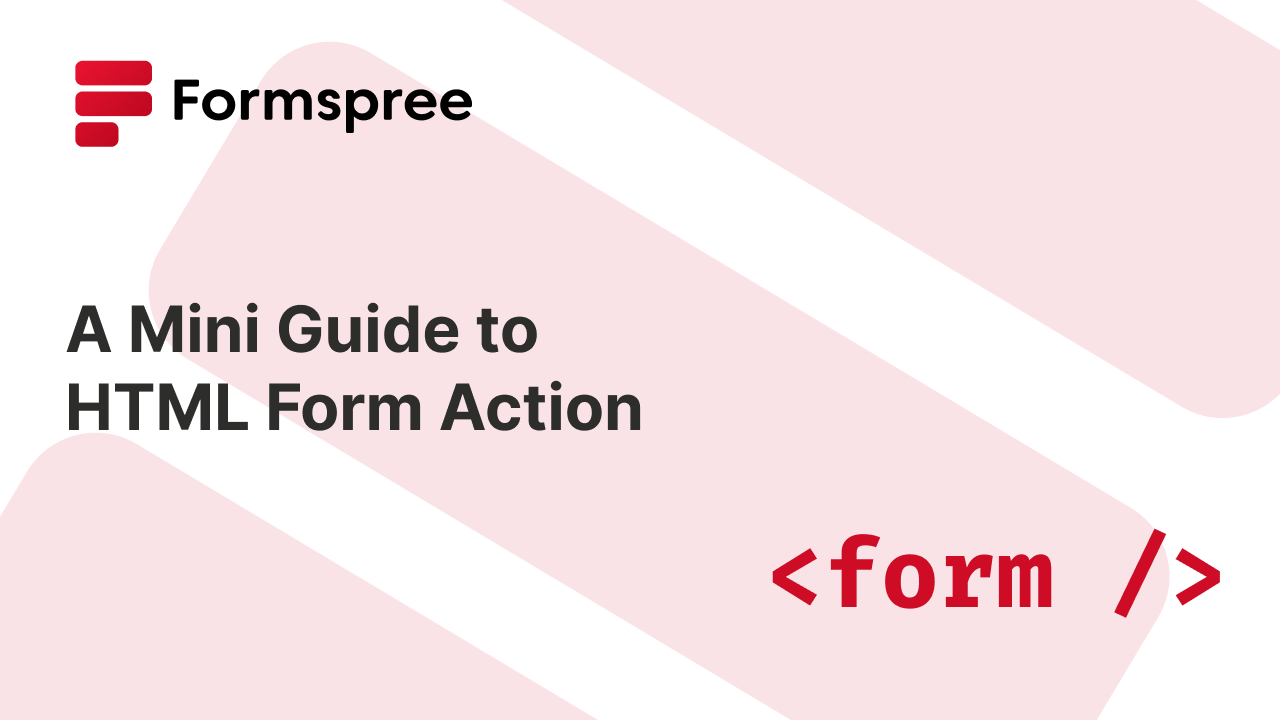
Forms are a cornerstone of the web, powering everything from contact pages to user registrations and checkout flows. In HTML, the <form>
element is the foundation for creating interactive forms. One of its most important attributes is action
, which determines where the form data is sent when a user submits it. Without properly understanding the action attribute, developers risk broken form submissions or unexpected behavior. This guide dives into the action attribute, explaining its syntax, use cases, and best practices to help you build functional and secure web forms.
What is the action Attribute?
The action attribute in an HTML <form> element
specifies the endpoint where form data should be sent upon submission. Its syntax is:
<form action="https://formspree.io/f/myzkjveq">
Here, the URL represents the target location, such as a server-side script or an API endpoint (or a forms backend, like Formspree). When a user submits the form, the browser packages the input data and sends it to the specified URL.
The main purpose of the action attribute is to define the destination for processing form submissions. For instance, a contact form might send data to a server script for sending an email, while a login form might route credentials to an authentication API.
Types of URLs for the action Attribute
The action attribute in HTML forms can accept two types of URLs: absolute and relative, each serving distinct purposes in web development.
Absolute URLs are complete URLs that specify the protocol, domain, and path. They are typically used to send form data to external servers or APIs. You might also need to use them if your site operates across multiple domains or subdomains, or if you are using a staging environment with different domains. Here’s how you use an absolute URL:
<form action="https://example.com/submit">
This URL ensures the form data is sent to a remote server, regardless of the current webpage’s location.
Relative URLs are relative to the current page or application’s domain and are commonly used within the same web application. For instance:
<form action="/submit">
This URL submits the form data to the /submit
route of the current domain.
You should use relative URLs when the forms backend is hosted on the same API as the frontend. They simplify maintenance and work well for internal routes. You should use absolute URLs when sending form data to external servers or APIs (e.g., https://formspree.io/f/myzkjveq). They are necessary for cross-domain submissions or integrations with third-party services.
Best Practices for Setting the action Attribute
When working with the form action attribute, following best practices ensures secure and efficient form submissions:
- Use Secure URLs: Always use HTTPS to protect data transmission to the web server. Whether it’s an absolute URL or a relative URL, prioritize security to avoid interception.
- Ensure Endpoint Compatibility: Verify that the target PHP file or web page can handle the input field data format (e.g.,
application/x-www-form-urlencoded
). - Use Descriptive Form Elements: Include clear labels like
<label for="lname">Last Name:</label>
to enhance usability. Combine them with the appropriate input elements, such as<input type="text">
, to ensure users can provide data seamlessly. - Test Form Action URLs: Ensure that the absolute URL or relative URL specified in the form action is valid and functional. Broken endpoints can disrupt user interactions.
- Error Handling: Set up error handling in your web server or PHP file to provide helpful feedback if submissions fail. For instance, confirm that the submit button (e.g.,
<input type="submit">
or<button type="submit">
) triggers a valid HTTP request.
The Relationship Between action and method
The action and method attributes work together to determine how form elements interact with a web server.
GET Method
When the method attribute is set to GET, the form sends data as a query string appended to the form action URL. This is useful for non-sensitive input elements like search forms. Example:
<form action="/action_page.php" method="GET">
<label for="lname">Last Name:</label>
<input type="text" name="lname">
<input type="submit" value="Search">
</form>
POST Method
With the post method, the input field data is sent in the HTTP request body. This is ideal for contact forms or when submitting sensitive information.
<form action="/action_page.php" method="POST">
<label for="lname">Last Name:</label>
<input type="text" name="lname">
<button type="submit">Submit</button>
</form>
Tip: Always test browser support for your form action URL and attribute values. Avoid using GET for sensitive data, and match the method attribute with the form’s purpose for optimal results.
Omitting the action Attribute
When the form action attribute is omitted in an HTML form, the browser defaults to submitting the form data to the same URL as the current web page. This behavior is often used in self-contained applications where the server processes and responds to the submitted data directly.
For example,
<form method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
In this case, the form elements like the text input will submit data using the specified HTTP method to the current page. While this can simplify form handling, it may cause issues if the page lacks the backend logic to handle the request. Always ensure the default value aligns with your application’s requirements.
Dynamic Actions
In some scenarios, you may need to set or modify the form action dynamically based on user input or conditions. This can be achieved using JavaScript, allowing your HTML form to handle multiple submission endpoints.
For example,
<form id="dynamicForm" method="POST">
<input type="text" id="name" name="name" placeholder="Enter your name">
<input type="submit" value="Submit">
</form>
<script>
const form = document.getElementById('dynamicForm');
form.onsubmit = () => {
form.action = form.name.value.includes('admin') ? '/admin' : '/user';
};
</script>
Setting the action value dynamically lets you adjust attribute values in real-time, enabling more flexible and responsive forms.
Debugging Common Issues
When an HTML form fails to work as expected, common problems include:
- Broken form action URL: Verify that the URL specified is correct and reachable.
- Incorrect HTTP method: Ensure the method (GET or POST) matches the server’s expectations.
- Invalid attribute values: Check for typos or unsupported settings for form elements.
- Browser Support: Ensure all input elements and methods are compatible with your target browsers.
Using developer tools, inspect the form’s submission behavior to troubleshoot issues effectively.
Conclusion
The form action attribute is an important part of HTML forms, defining where form data is sent and ensuring smooth interaction between users and web servers. By understanding its syntax, possible values, and best practices, you can create secure and functional forms tailored to your application’s needs. And whether you’re using static or dynamic actions, always test and validate your form elements to handle different scenarios and ensure compatibility across browsers.